Compose email in your way!
This is a very interesting app which lets you compose the email in a different way if you don't want to edit it in the Android's default Email app.
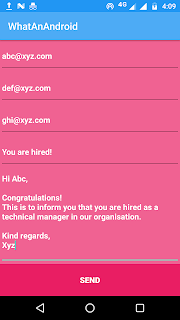
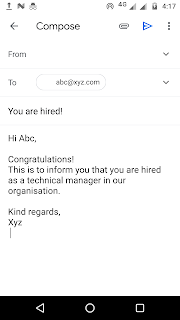
This is a very interesting app which lets you compose the email in a different way if you don't want to edit it in the Android's default Email app.
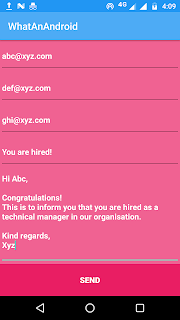
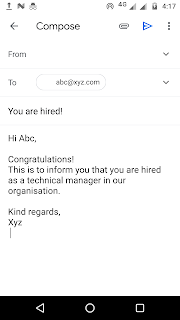
---------------------------------------- email_activity.xml ---------------------------------------
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="#F06292"
android:gravity="center|top">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="8"
android:orientation="vertical">
<EditText
android:id="@+id/ea_toET"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="left|center"
android:textStyle="bold"
android:hint="To"
android:textColorHint="#cdcdcd"
android:textColor="#ffffff"
android:textSize="16sp"/>
<EditText
android:id="@+id/ea_ccET"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="left|center"
android:textStyle="bold"
android:hint="CC"
android:textColorHint="#cdcdcd"
android:textColor="#ffffff"
android:textSize="16sp"/>
<EditText
android:id="@+id/ea_bccET"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="left|center"
android:textStyle="bold"
android:hint="BCC"
android:textColorHint="#cdcdcd"
android:textColor="#ffffff"
android:textSize="16sp"/>
<EditText
android:id="@+id/ea_subjectET"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="left|center"
android:textStyle="bold"
android:hint="Subject"
android:textColorHint="#cdcdcd"
android:textColor="#ffffff"
android:textSize="16sp"/>
<EditText
android:id="@+id/ea_messageET"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="3"
android:gravity="left|top"
android:textStyle="bold"
android:hint="Message"
android:textColorHint="#cdcdcd"
android:textColor="#ffffff"
android:textSize="16sp"/>
<Button
android:id="@+id/ea_sendBTN"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="center"
android:textStyle="bold"
android:text="Send"
android:background="#E91E63"
android:textColor="#ffffff"
android:textSize="16sp"/>
</LinearLayout>
</LinearLayout>
---------------------------------------------------------------
-------------------------------------- EmailActivity.java ------------------------------------
package com.app.yourappname;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
public class EmailActivity extends AppCompatActivity {
private TextView toET, ccET, bccET, subjectET, msgET;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.email_activity);
Button sendBTN;
toET = findViewById(R.id.ea_toET);
ccET = findViewById(R.id.ea_ccET);
bccET = findViewById(R.id.ea_bccET);
subjectET = findViewById(R.id.ea_subjectET);
msgET = findViewById(R.id.ea_messageET);
sendBTN = findViewById(R.id.ea_sendBTN);
sendBTN.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if(toET.getText().toString().equals("")) {
Toast.makeText(EmailActivity.this, "You forgot to write recipient's email address...", Toast.LENGTH_LONG).show();
return;
}
sendElectronicMain();
}
});
}
private void sendElectronicMain() {
Intent emailIntent = new Intent(android.content.Intent.ACTION_SEND);
String[] recipients = new String[]{toET.getText().toString()};
emailIntent.putExtra(android.content.Intent.EXTRA_EMAIL, recipients);
emailIntent.putExtra(android.content.Intent.EXTRA_CC, ccET.getText().toString());
emailIntent.putExtra(Intent.EXTRA_BCC, bccET.getText().toString());
emailIntent.putExtra(android.content.Intent.EXTRA_SUBJECT, subjectET.getText().toString());
String emailMsg = new String(msgET.getText().toString());
emailIntent.putExtra(android.content.Intent.EXTRA_TEXT, emailMsg);
emailIntent.setType("plain/text");
startActivity(emailIntent);
}
}
---------------------------------------------------------------------
----------------------------------- Declare Activity name in Manifest file ---------------------------
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.app.yourappname">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".EmailActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
---------------------------------------------------------------------
No comments:
Post a Comment